⏰문제
스도쿠는 숫자퍼즐로, 가로 9칸 세로 9칸으로 이루어져 있는 표에 1 부터 9 까지의 숫자를 채워넣는 퍼즐이다.
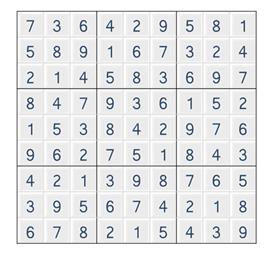
같은 줄에 1 에서 9 까지의 숫자를 한번씩만 넣고, 3 x 3 크기의 작은 격자 또한, 1 에서 9 까지의 숫자가 겹치지 않아야 한다.
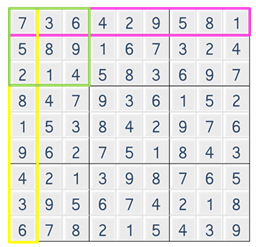
입력으로 9 X 9 크기의 스도쿠 퍼즐의 숫자들이 주어졌을 때, 위와 같이 겹치는 숫자가 없을 경우, 1을 정답으로 출력하고 그렇지 않을 경우 0 을 출력한다.
[제약 사항]
1. 퍼즐은 모두 숫자로 채워진 상태로 주어진다.
2. 입력으로 주어지는 퍼즐의 모든 숫자는 1 이상 9 이하의 정수이다.
[입력]
입력은 첫 줄에 총 테스트 케이스의 개수 T가 온다.
다음 줄부터 각 테스트 케이스가 주어진다.
테스트 케이스는 9 x 9 크기의 퍼즐의 데이터이다.
[출력]
테스트 케이스 t에 대한 결과는 “#t”을 찍고, 한 칸 띄고, 정답을 출력한다.
(t는 테스트 케이스의 번호를 의미하며 1부터 시작한다.)
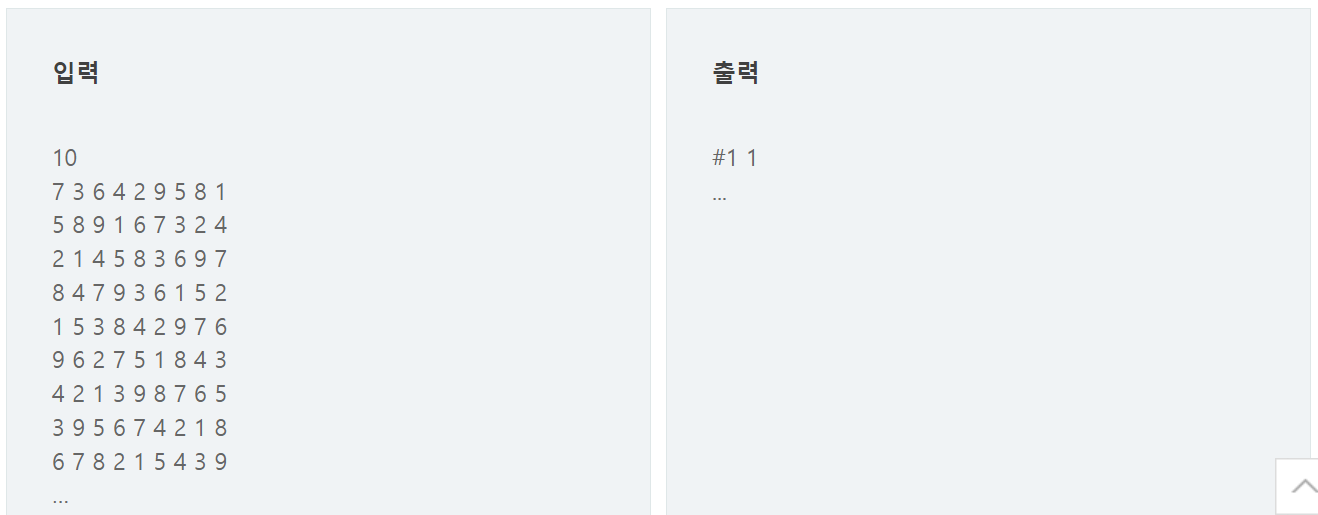
⌨️코드
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
|
import java.util.Arrays;
import java.util.Scanner;
public class exp_1974 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int T=sc.nextInt();
for(int test_case = 1; test_case <= T; test_case++)
{
int size = 9;
int[][] arr = new int[size][size];
for(int i=0;i<size;i++) {//9*9배열 생성
for(int j=0;j<size;j++) {
arr[i][j] = sc.nextInt();
}
}
// 가로 검사
int result = 1;
for(int i=0;i<size;i++) {
int[] count = new int[10]; //0~8까지. 새로운 가로 시작하면 초기화
for(int j=0;j<size;j++) {
count[arr[i][j]]++;
if(count[arr[i][j]]>1) {
result = 0;
break;
}
}// j for문 끝
//System.out.println(Arrays.toString(count));
}// i for문 끝
//System.out.println(result);
// 세로 검사
for(int i=0;i<size;i++) {
int[] count = new int[10]; //0~8까지. 새로운 가로 시작하면 초기화
for(int j=0;j<size;j++) {
count[arr[j][i]]++;
if(count[arr[j][i]]>1) {
result = 0;
break;
}
}// j for문 끝
//System.out.println(Arrays.toString(count));
}// i for문 끝
//System.out.println(result);
// 정사각형 검사
for(int i=0;i<=6;i+=3) {
for(int j=0;j<=6;j+=3) {
int[] count = new int[10];
for(int k=i;k<i+3;k++) {
for(int l=j;l<j+3;l++) {
count[arr[k][l]]++;
//System.out.println(k+","+l);
if(count[arr[k][l]]>1) {
result = 0;
break;
}
}
}//System.out.println(Arrays.toString(count));
}
}
//System.out.println(result);
System.out.println("#"+test_case+" "+result);
}
}
}
|
cs |
'⚙️알고리즘' 카테고리의 다른 글
백준) 10828 : 스택 (0) | 2023.10.28 |
---|---|
백준) 2573 : 빙산 (0) | 2023.10.28 |
[solved.ac]1000.a+b (0) | 2023.02.19 |
[SWE]1966. 숫자를 정렬하자 (0) | 2023.02.15 |
[SWE]2068. 최대수 구하기 (0) | 2023.02.15 |